The Essential Guide to Testing Node.js Applications
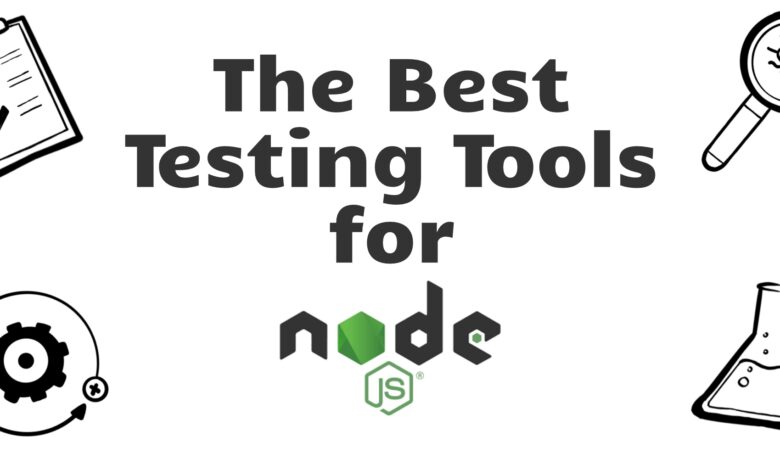
In the realm of Node.js development, ensuring the reliability and robustness of your applications is crucial. Testing plays a pivotal role in achieving this goal, helping developers identify and rectify issues early in the development process. In this comprehensive guide, we will explore the key aspects of testing Node.js applications, from setting up a testing environment to implementing effective testing strategies.
- Understanding the Basics of Node.js Testing
The Importance of Testing in Node.js:
Testing is not just about finding bugs; it’s a proactive approach to building resilient applications. In Node.js development, where asynchronous and event-driven paradigms are prevalent, comprehensive testing ensures that your application behaves predictably under various conditions.
Leveraging Mocha and Jest:
Mocha and Jest are popular testing frameworks for Node.js. While Mocha provides flexibility with various assertion libraries, Jest, developed by Facebook, offers an integrated testing solution with built-in assertions. Choosing the right framework depends on your project requirements and preferences.
- Setting Up a Testing Environment
Environment Configuration:
Establishing a dedicated testing environment is essential. This includes configuring a separate database for testing, managing environment variables, and ensuring that your testing environment mimics the production environment as closely as possible.
Docker for Consistency:
Consider using Docker for containerization to create consistent testing environments across different development machines. Docker ensures that dependencies are isolated, minimizing the “it works on my machine” problem.
- Writing Unit Tests for Node.js Code
The Essence of Unit Testing:
Unit tests focus on individual units of code, verifying that each function or method behaves as expected. In Node.js, unit tests are essential for validating the logic and functionality of your code in isolation.
Test-Driven Development (TDD):
Adopting a Test-Driven Development approach can enhance the effectiveness of unit testing. Write tests before writing the actual code, clarifying the expected behavior and encouraging modular, testable code.
- Integration Testing in Node.js Applications
Testing the Interaction of Components:
Integration testing goes beyond unit testing and evaluates the interactions between different components of your application. In Node.js, where modules and external services are interconnected, integration tests validate the seamless collaboration of these elements.
Supertest for API Testing:
When testing APIs in Node.js, Supertest is a valuable library. It allows you to make HTTP requests and assert the responses, enabling thorough testing of your API endpoints.
- End-to-End (E2E) Testing with Node.js
Simulating Real User Interactions:
End-to-End testing ensures that your application functions as expected from a user’s perspective. Tools like Puppeteer enable you to automate browser interactions, mimicking real user behavior.
Cypress for Modern E2E Testing:
Consider using Cypress for E2E testing. Cypress provides a fast, reliable, and easy-to-use testing framework with real-time feedback during test execution. Its interactive test runner and ability to stub network requests make it a favorite among developers.
- Mocking in Node.js Tests
Creating Controlled Test Environments:
Mocking involves creating controlled environments for your tests by simulating external dependencies. In Node.js, where external services like databases and APIs are common, mocking ensures that tests remain isolated and predictable.
Sinon.js for Comprehensive Mocking:
Sinon.js is a versatile library for mocking, spying, and stubbing in Node.js tests. Its rich feature set includes the ability to create spies to monitor function calls, stub functions to control their behavior, and mock timers for testing time-dependent functionality.
- Continuous Integration (CI) for Node.js Testing
Automating Testing Workflows:
Integrating testing into your continuous integration workflow is essential for catching issues early in the development pipeline. CI tools like Jenkins, Travis CI, or GitHub Actions can automatically run tests whenever code changes are pushed.
GitHub Actions for Seamless Integration:
GitHub Actions, directly integrated into GitHub repositories, offers a seamless CI/CD solution for Node.js projects. Define your workflows using YAML and trigger tests on each pull request, ensuring that changes meet quality standards before merging.
- Performance Testing in Node.js
Evaluating Scalability and Response Times:
Performance testing in Node.js involves assessing how well your application handles different levels of load and stress. Tools like Artillery and Apache JMeter can simulate concurrent users, helping you identify and address performance bottlenecks.
Load Testing with Artillery:
Artillery is a powerful, open-source load testing toolkit for Node.js applications. With a simple YAML configuration, you can define scenarios and stress-test your APIs, WebSocket connections, or any HTTP-based services.
- Security Testing Practices
Identifying and Mitigating Vulnerabilities:
Security testing is crucial for identifying vulnerabilities and ensuring that your Node.js application is resistant to common security threats. Tools like ESLint for code analysis and OWASP ZAP for penetration testing contribute to a more secure codebase.
npm Audit for Dependency Security:
Leverage npm audit to automatically scan your project’s dependencies for known security vulnerabilities. Regularly running npm audit ensures that your application stays protected against potential exploits.
- Documentation and Best Practices
Documenting Your Testing Strategies:
Comprehensive documentation is essential for maintaining a clear understanding of your testing strategies. Document the purpose of each test suite, the intended coverage, and any specific configurations to facilitate collaboration within your development team.
Share Testing Insights in Onboarding:
During the onboarding process, share insights into your testing strategies with new team members. This ensures a consistent approach to testing practices and empowers developers to contribute effectively to the testing efforts.
Conclusion
In conclusion, testing Node.js applications is a multifaceted endeavor that requires a strategic approach. From unit tests to end-to-end testing and beyond, each testing type contributes to the overall resilience and reliability of your application.
As you strengthen your development team, consider the strategic decision to hire Node.js developers, ensuring expertise in testing and quality assurance. Simultaneously, for a specialized focus on testing practices, it’s advantageous to hire QA engineer. Their combined skills contribute to the creation of a robust and secure Node.js application, elevating your testing practices in the dynamic landscape of software development.